Cypress Tutorial
Cypress Hooks
What Are Cypress Hooks ?
Cypress hooks are the block of codes that run pre/post of the test cases.
Most common Cypress hooks are :
before() - runs once before first test case of a suite. Syntax :
before(function() {
// steps
})
OR
before(function functionName() {
// steps
})
OR
before('description' function() {
// steps
})
after() - runs once after last test case of a suite. Syntax :
after(function() {
// steps
})
OR
after(function functionName() {
// steps
})
OR
after('description' function() {
// steps
})
beforeEach() - runs before each test case of a suite. Syntax :
beforeEach(function() {
// steps
})
OR
beforeEach(function functionName() {
// steps
})
OR
beforeEach('description' function() {
// steps
})
afterEach() - runs after each test case of a suite. Syntax :
afterEach(function() {
// steps
})
OR
afterEach(function functionName() {
// steps
})
OR
afterEach('description' function() {
// steps
})
To understand its hierarchy, consider below example :
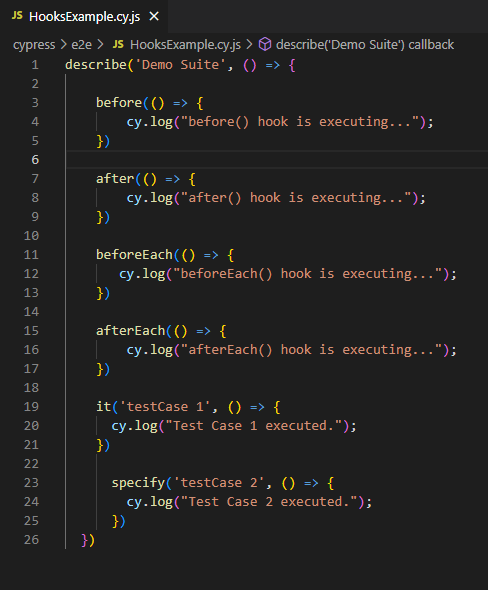
Ouput will be :
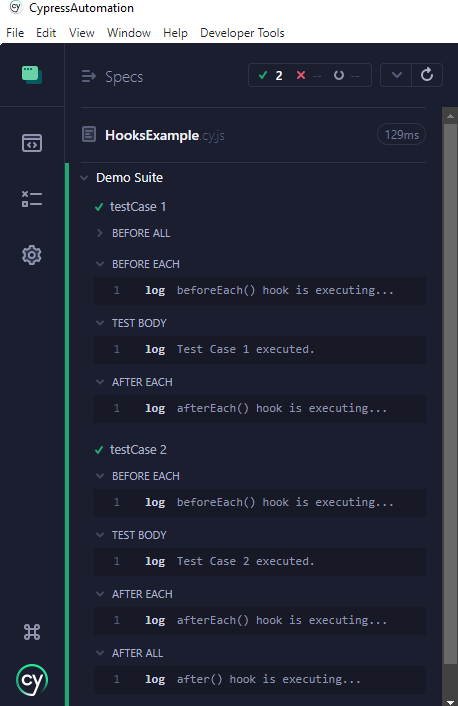
We can construct test suite using describe() or context().
And test cases can be created with the help of it() or specify().
How to Include and Exclude a Test Case or a Test Suite ?
Cypress provides specific keywords to include or exclude the suite and test cases.
To include a test case or a test suite .only keyword is used. The syntax :
For test suite -
describe.only('Test Suite 1', () => {
// steps
})
For test case -
it.only('Test case 1', () => {
// steps
})
For exlusion of a test case or a test suite, cypress provides .skip keyword.
For test suite -
describe.skip('Test Suite 1', () => {
// steps
})
For test case -
it.skip('Test case 1', () => {
// steps
})